# FAQ
The FAQ module contains a single FAQ, FAQ section and all FAQ categories, and supports different ways to jump to robot and human services through configuration.
# API
# showAllFAQSections()
You can call this function to display the FAQ section list, you can search a specific FAQ, and show the entrance of AIHelp conversation system in the appropriate location:
AIHelpSupport::showAllFAQSections();
Or, you could customize the FAQ module as you need:
ConversationConfig faqConversationConfig = ConversationConfigBuilder()
.setConversationIntent(ConversationIntent::BOT_SUPPORT)
.setAlwaysShowHumanSupportButtonInBotPage(true)
.setWelcomeMessage("This is a welcome message for FAQ.")
.build();
FaqConfig config = FaqConfigBuilder()
.setShowConversationMoment(ShowConversationMoment::ALWAYS)
.setConversationConfig(faqConversationConfig)
.build();
AIHelpSupport::showAllFAQSections(config);
# showFAQSection()
Different from displaying all FAQ sections, AIHelp supports displaying a FAQ section separately in some special scenarios:
AIHelpSupport::showFAQSection("SECTION ID")
Or, you could customize the API as you need:
ConversationConfig faqConversationConfig = ConversationConfigBuilder()
.setConversationIntent(ConversationIntent::BOT_SUPPORT)
.setAlwaysShowHumanSupportButtonInBotPage(true)
.setWelcomeMessage("This is a welcome message for FAQ.")
.build();
FaqConfig config = FaqConfigBuilder()
.setShowConversationMoment(ShowConversationMoment::ONLY_IN_ANSWER_PAGE)
.setConversationConfig(faqConversationConfig)
.build();
AIHelpSupport.showFAQSection("SECTION ID", config);
# showSingleFAQ()
You can show a specific FAQ question by calling this method:
AIHelpSupport::showSingleFAQ("FAQ ID")
Or, you could customize the API as you need:
ConversationConfig faqConversationConfig = ConversationConfigBuilder()
.setConversationIntent(ConversationIntent::BOT_SUPPORT)
.setAlwaysShowHumanSupportButtonInBotPage(true)
.setWelcomeMessage("This is a welcome message for FAQ.")
.build();
FaqConfig config = FaqConfigBuilder()
.setShowConversationMoment(ShowConversationMoment::AFTER_MARKING_UNHELPFUL)
.setConversationConfig(faqConversationConfig)
.build();
AIHelpSupport::showSingleFAQ("FAQ ID", config);
# Definition
# faqConfig
- Type:
FaqConfig
- Default:
null
- Details: Configuration for FAQ module
# showConversationMoment
- Type:
ShowConversationMoment
- Default:
showConversationMoment::NEVER
- Details: when to show conversation entrance in FAQ page, always / never / after-marking-unhelpful / only-in-answer-page
# conversationConfig
- Type:
ConversationConfig
- Default:
null
- Detail: Configuration for customer service
# conversationIntent
- Type:
ConversationIntent
- Default:
ConversationIntent::BOT_SUPPORT
- Detail: Intent for customer service display
# alwaysShowHumanSupportButtonInBotPage
- Type:
bool
- Default:
false
- Detail: Entrance visibility for human support button in bot page
# welcomeMessage
- Type:
string
- Default:
''
- Detail: Custom welcome message for human support
# storyNode
- Type:
string
- Default:
''
- Detail: Entrance node for specific story line, a.k.a: story lines' user say
# sectionId
- Type:
string
- Default:
''
- Details: The ID of the FAQ section about to display, you can get it at here:
# faqId
- Type:
string
- Default:
''
- Details: The ID of the specific FAQ about to display, you can get it at here:
# Scenario
Let's assume a scenario.
A season is finally over. You configure a series of Season award related issues in the AIHelp dashboard, and then show them on the first screen of the game by FAQ, hoping to solve the problems that users may have.
At the same time, the game has different support and care schemes for different levels of users.
The details are as follows:
1、Users with level < 20 can only see the conversation entrance button after marking the FAQ unhelpful;
2、Users with level 20 < level < 50 can always see the conversation entrance button in the upper right corner of the FAQ page. After clicking this button, users can contact robot customer service by default. At the same time, the access to contact manual customer service is provided in the upper right corner of the robot page;
3、Users with level > 50 can always see the contact customer service button on the FAQ page. After clicking, they can directly contact manual customer service and display a special welcome message different from other users.
Then, the code example for this scenario is as follows:
public void showAllFAQSections(int level) {
FaqConfigBuilder faqBuilder = new FaqConfigBuilder();
ConversationConfigBuilder conversationBuilder = new ConversationConfigBuilder();
if (level < 20) {
faqBuilder.setShowConversationMoment(ShowConversationMoment::AFTER_MARKING_UNHELPFUL);
} else if (level < 50) {
faqBuilder.setShowConversationMoment(ShowConversationMoment::ALWAYS);
conversationBuilder.setAlwaysShowHumanSupportButtonInBotPage(true);
faqBuilder.setConversationConfig(conversationBuilder.build());
} else {
faqBuilder.setShowConversationMoment(ShowConversationMoment::ALWAYS);
conversationBuilder.setConversationIntent(ConversationIntent::HUMAN_SUPPORT);
conversationBuilder.setWelcomeMessage("THIS IS YOUR SPECIAL WELCOME MESSAGE");
faqBuilder.setConversationConfig(conversationBuilder.build());
}
AIHelpSupport::showAllFAQSections(faqBuilder.build());
}
# Page Example
Page examples based on the above scenario are as follows:
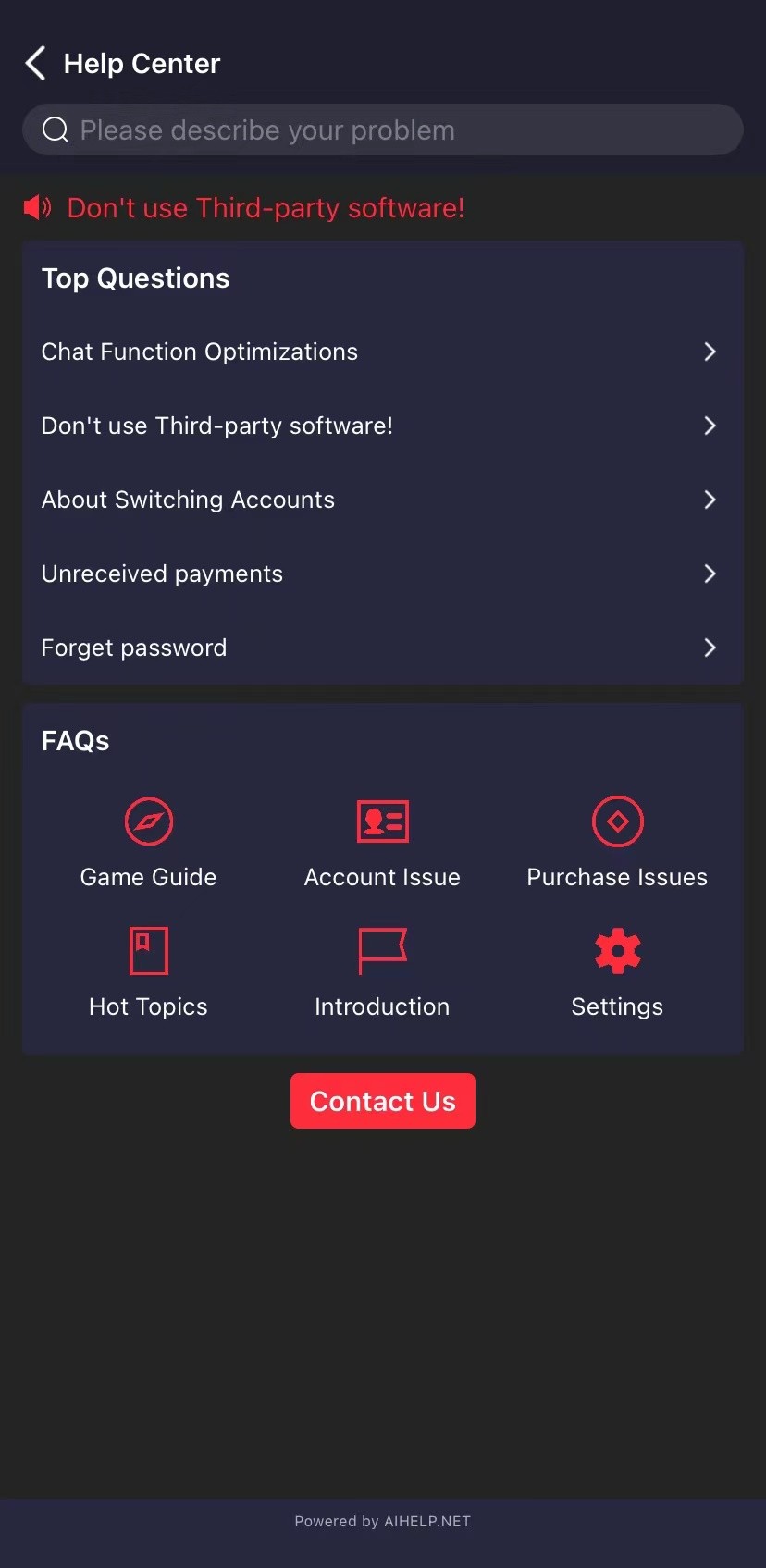
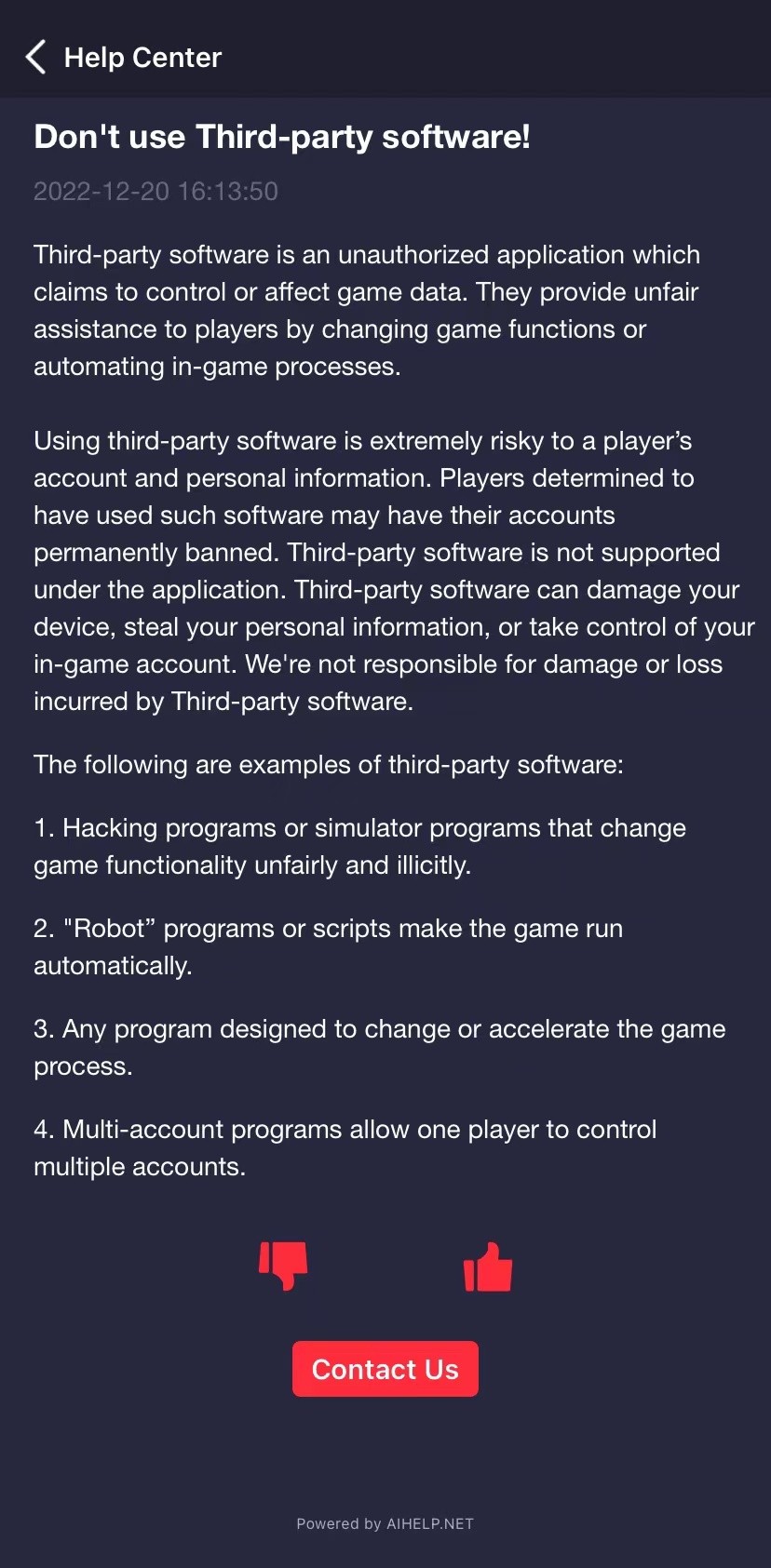
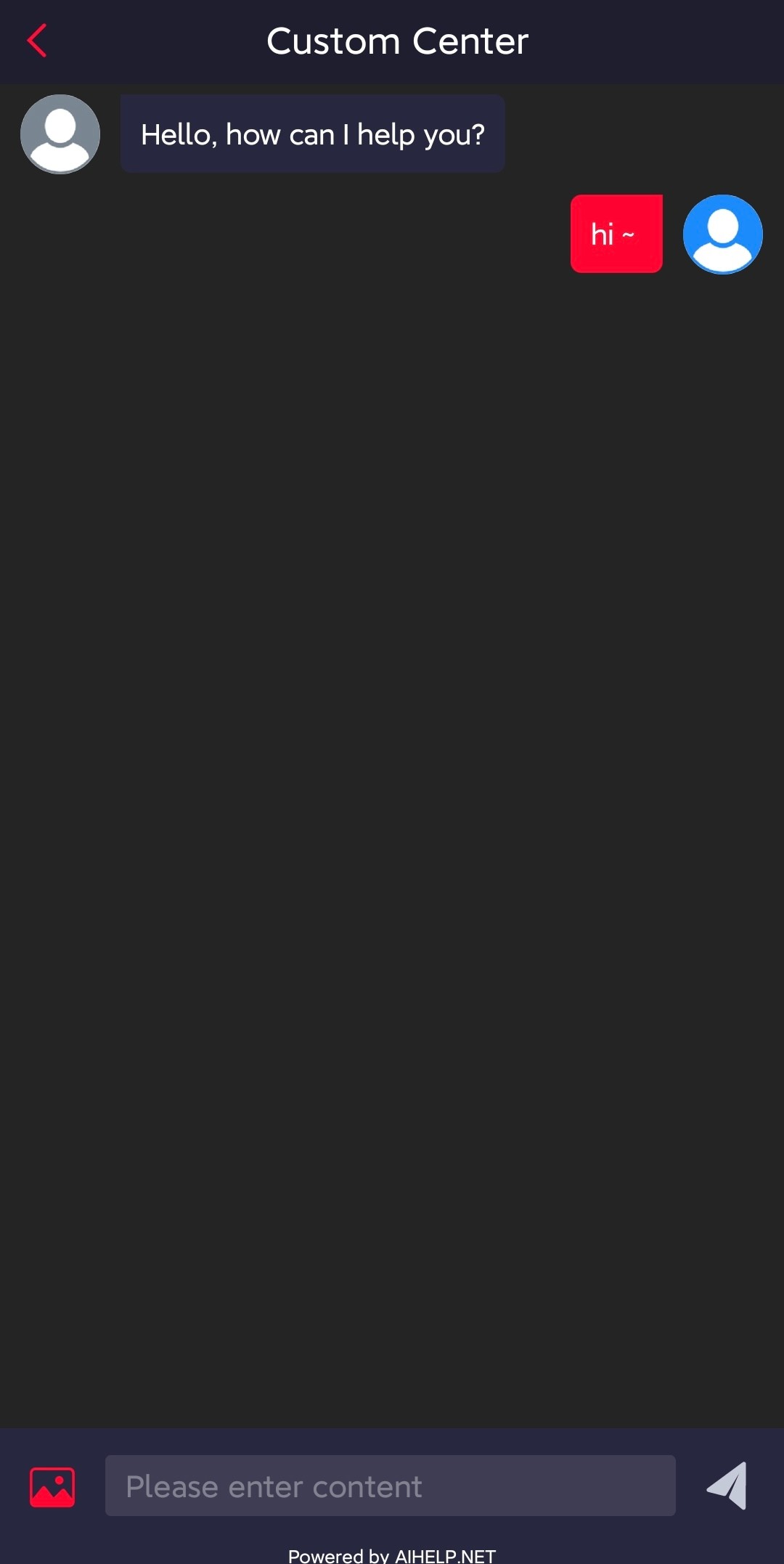