# Operation
We integrate announcements, guides, news, etc. with the customer service system at the same entrance, greatly improving the efficiency of information dissemination, so that users can understand the latest product trends more conveniently, and communicate with the customer service team more directly and efficiently.
# API
# showOperation()
You can call the AIHelp operation module by calling this function:
AIHelpSupport::showOperation();
Or, you could customize the Operation module as you need:
ConversationConfig operationConversationConfig = ConversationConfigBuilder()
.setAlwaysShowHumanSupportButtonInBotPage(true)
.setWelcomeMessage("This is a welcome message for operation.")
.build();
OperationConfig config = OperationConfigBuilder()
.setConversationTitle("Hi, there")
.setSelectIndex(2)
.setConversationConfig(operationConversationConfig)
.build();
AIHelpSupport::showOperation(config);
# Definition
# operationConfig
- Type:
OperationConfig
- Default:
null
- Details: Configuration for Operation module
# conversationConfig
- Type:
ConversationConfig
- Default:
null
- Details: Configuration for customer service comes from Operation module
# selectIndex
- Type:
int
- Default:
0
- Details: Default select tab index in operation
# conversationTitle
- Type:
string
- Default:
HELP
- Details: The tab title for customer service in operation
# conversationIntent
- Type:
ConversationIntent
- Default:
ConversationIntent::BOT_SUPPORT
- Details: Intent for customer service display
# alwaysShowHumanSupportButtonInBotPage
- Type:
bool
- Default:
false
- Details: Entrance visibility for human support button in bot page
# welcomeMessage
- Type:
string
- Default:
''
- Details: Custom welcome message for human support
# storyNode
- Type:
string
- Default:
''
- Details: Entrance node for specific story line, a.k.a: story lines' user say
# Scenario
Let’s assume a scenario where the application has different support and care schemes for different levels of users.
1、For users with level > 50, conversation tab is selected by default, and configure their conversation title to "Support";
2、At the same time, these users can choose to contact the manual customer service directly, and there is a special welcome message for them.
Then, the code example for this scenario is as follows:
public void showOperation(int level) {
OperationConfigBuilder operationBuilder = new OperationConfigBuilder();
if (level > 50) {
operationBuilder.setSelectIndex(Integer.MAX_VALUE);
operationBuilder.setConversationTitle("Support");
operationBuilder.setConversationConfig(new ConversationConfigBuilder()
.setAlwaysShowHumanSupportButtonInBotPage(true)
.setWelcomeMessage("CUSTOM WELCOME MSG FROM OPERATION")
.build()
);
} else {
operationBuilder.setSelectIndex(0);
}
AIHelpSupport.showOperation(operationBuilder.build());
}
# Page Example
Page examples based on the above scenario are as follows:
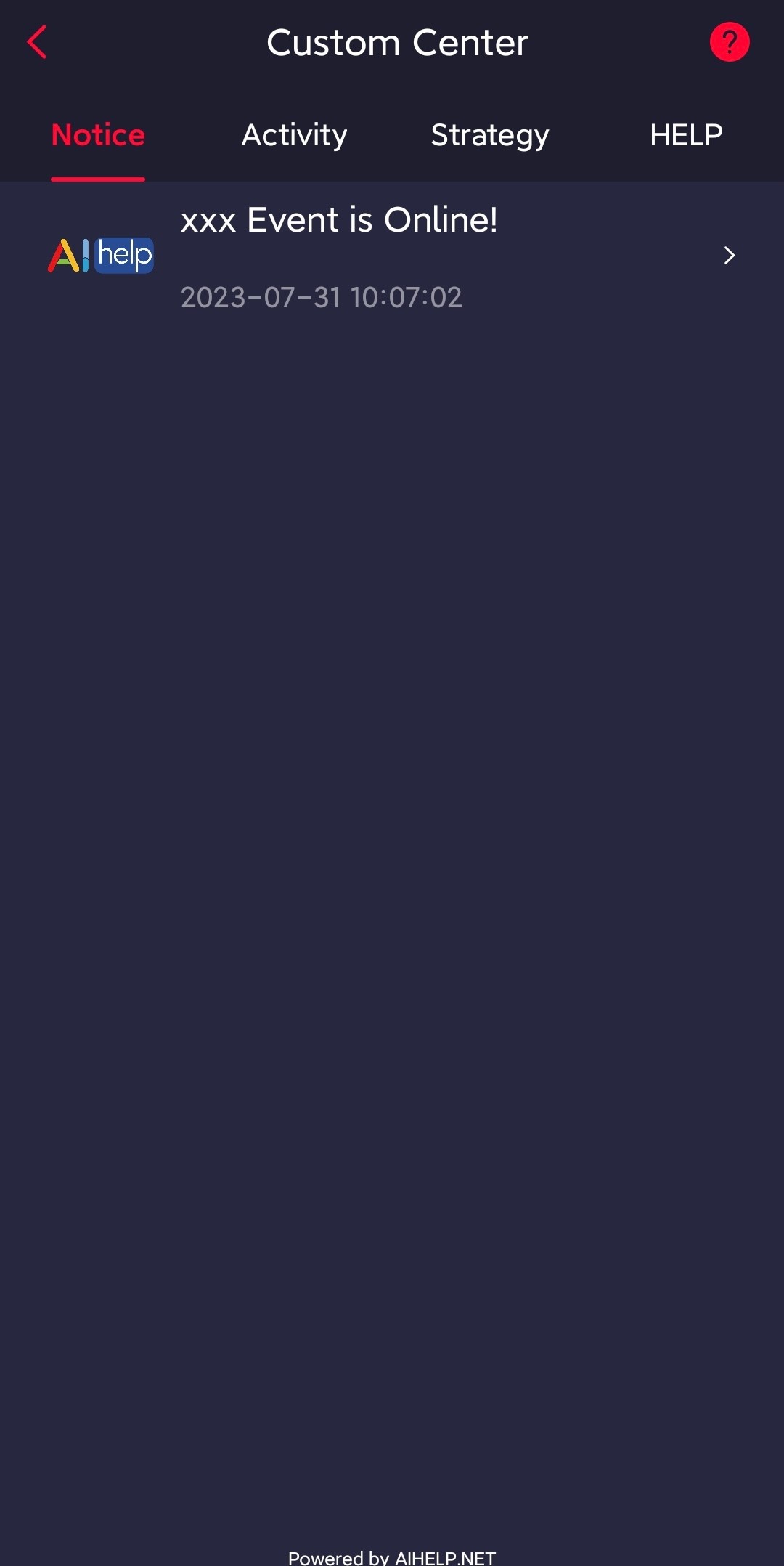
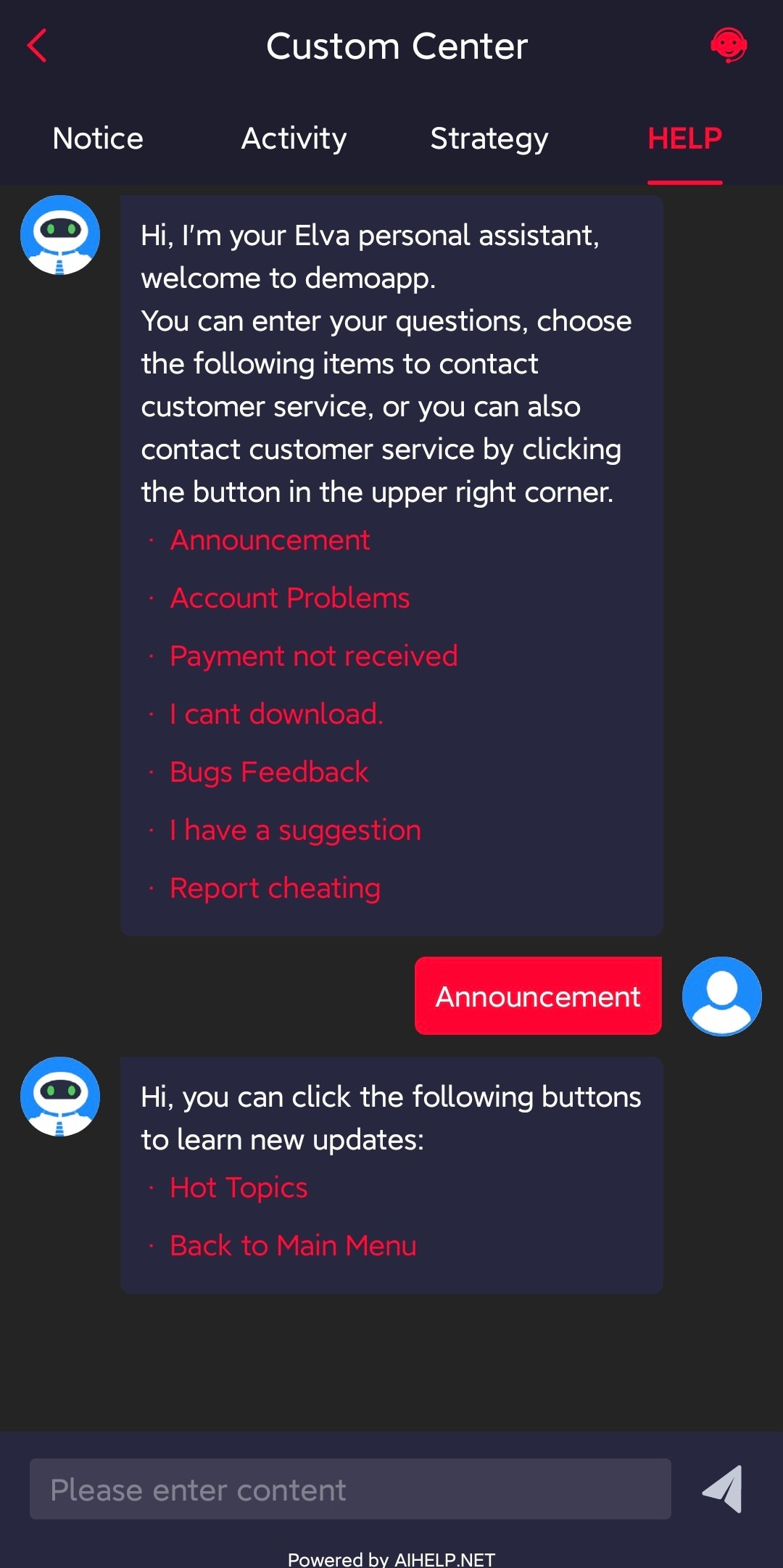
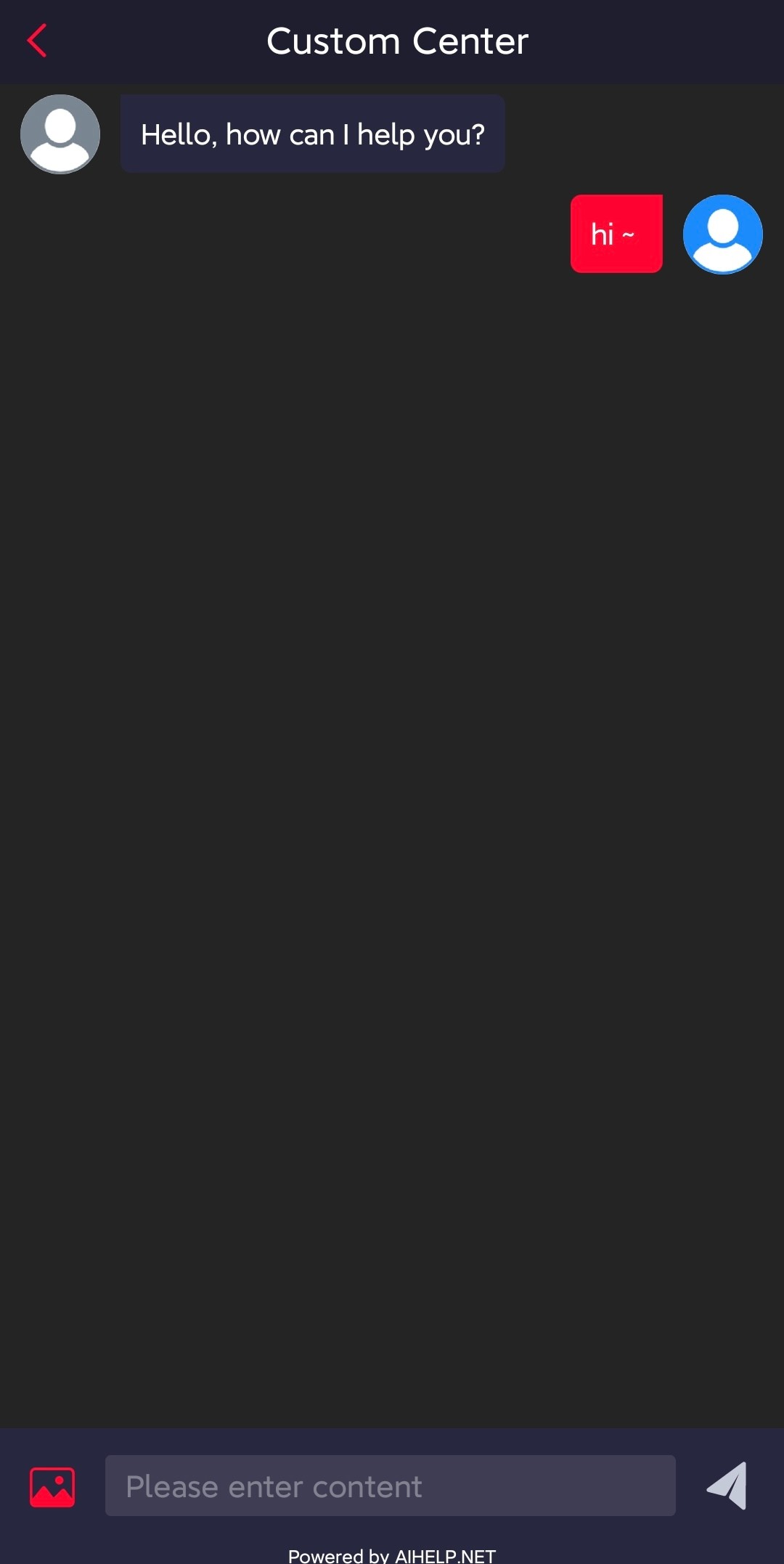