# FAQ
The FAQ module contains a single FAQ, FAQ section and all FAQ categories, and supports different ways to jump to robot and human services through configuration.
# API
You could set the supportMode
property as showAllFAQSections
, showFAQSection
or showSingleFAQ
to notify AIHelp that you want to display the FAQ page:
<script src="https://cdn.aihelp.net/webchatv3/aihelp.js"></script>
<body>
<script>
(function () {
let initConfig = {
appKey: "THIS IS YOUR APP KEY",
domain: "THIS IS YOUR APP DOMAIN",
appId: "THIS IS YOUR APP ID",
supportMode: 'showAllFAQSections'
};
AIHelpSupport.init(initConfig);
AIHelpSupport.show();
})();
</script>
</body>
Or, you could make your own configuration about AIHelp via the supportConfig
property like this:
<script src="https://cdn.aihelp.net/webchatv3/aihelp.js"></script>
<body>
<script>
(function () {
let initConfig = {
appKey: "THIS IS YOUR APP KEY",
domain: "THIS IS YOUR APP DOMAIN",
appId: "THIS IS YOUR APP ID",
supportMode: 'showSingleFAQ',
supportConfig: {
conversationIntent: 'human',
welcomeMessage: 'This is a welcome message for FAQ',
showConversationMoment: 'always',
faqId: 'THIS IS YOUR FAQ ID'
}
};
AIHelpSupport.init(initConfig);
AIHelpSupport.show();
})();
</script>
</body>
# URL
Or, you could implement the same function by the URL scheme:
<script>
let appKey = "THIS IS YOUR APP KEY";
let domain = "THIS IS YOUR APP DOMAIN";
let appId = "THIS IS YOUR APP ID";
let faqId = "THIS IS YOUR FAQ ID";
(function () {
let baseUrl = `https://${domain}/webchatv3/#/appKey/${appKey}/domain/${domain}/appId/${appId}`;
let params = `supportMode=showSingleFAQ&faqId=${faqId}&showConversationMoment=always&conversationIntent=human`;
let url = `${baseUrl}?${params}`;
window.open(url);
})();
</script>
# Definition
# supportMode
- Type:
showAllFAQSections | showFAQSection | showSingleFAQ
- Detail: Required. Support mode for AIHelp,
showAllFAQSections
for all FAQ sections,showFAQSection
for a specific FAQ section, andshowSingleFAQ
for a specific FAQ.
# supportConfig API
- Type:
object
- Default:
{}
- Detail: Optional. Only works in API, customize configuration for AIHelp.
# conversationIntent
- Type:
bot | human
- Default:
bot
- Detail: Optional. Intent for customer service display,
bot
for robot service,human
for human service.
# alwaysShowHumanSupportButtonInBotPage
- Type:
boolean
- Default:
false
- Detail: Optional. Entrance visibility for human support button in bot page.
# welcomeMessage
- Type:
string
- Default:
''
- Detail: Optional. Custom welcome message for human support.
# storyNode
- Type:
string
- Default:
''
- Detail: Optional. Entrance node for specific story line, a.k.a: story lines' user say.
# showConversationMoment
- Type:
always | never | onlyInAnswerPage | afterMarkingHelpful
- Default:
never
- Detail: Optional. When to show a customer service entrance in FAQ page.
# sectionId showFAQSection
- Type:
string
- Detail: Required. Only works when setting
supportMode
asshowFAQSection
. You can get it here:
# faqId showSingleFAQ
- Type:
string
- Detail: Required. Only works when setting
supportMode
asshowSingleFAQ
. You can get it here:
# Scenario
Let's assume a scenario.
A season is finally over. You configure a series of Season award related issues in the AIHelp dashboard, and then show them on the first screen of the game by FAQ, hoping to solve the problems that users may have.
At the same time, the game has different support and care schemes for different levels of users.
The details are as follows:
1、Users with level < 20 can only see the conversation entrance button after marking the FAQ unhelpful;
2、Users with level 20 < level < 50 can always see the conversation entrance button in the upper right corner of the FAQ page. After clicking this button, users can contact robot customer service by default. At the same time, the access to contact manual customer service is provided in the upper right corner of the robot page;
3、Users with level > 50 can always see the contact customer service button on the FAQ page. After clicking, they can directly contact manual customer service and display a special welcome message different from other users.
Then, the code example for this scenario is as follows:
# API
<script src="https://cdn.aihelp.net/webchatv3/aihelp.js"></script>
<script>
(function (level) {
let supportConfig = {};
if (level < 20) {
supportConfig["showConversationMoment"] = 'afterMarkingHelpful';
} else if (level < 50) {
supportConfig["showConversationMoment"] = 'always';
supportConfig["alwaysShowHumanSupportButtonInBotPage"] = true;
} else {
supportConfig["showConversationMoment"] = 'always';
supportConfig["conversationIntent"] = 'human';
supportConfig["welcomeMessage"] = "This is a welcome message for FAQ";
}
var initConfig = {
appKey: "THIS IS YOUR APP KEY",
domain: "THIS IS YOUR APP DOMAIN",
appId: "THIS IS YOUR APP ID",
appName: "THIS IS YOUR APP NAME(OPTIONAL)",
language: "THIS IS YOUR DEFAULT LANGUAGE(OPTIONAL)",
supportMode: "showAllFAQSections",
supportConfig: supportConfig,
};
AIHelpSupport.init(initConfig);
AIHelpSupport.show();
})();
</script>
# URL
<script>
let appKey = 'THIS IS YOUR APP KEY';
let domain = 'THIS IS YOUR APP DOMAIN';
let appId = 'THIS IS YOUR APP ID';
(function (level) {
let url = `https://${domain}/webchatv3/#/appKey/${appKey}/domain/${domain}/appId/${appId}?supportMode=showAllFAQSections`;
if (level < 20) {
url = `${url}&showConversationMoment=afterMarkingHelpful`;
} else if (level < 50) {
url = `${url}&showConversationMoment=always&alwaysShowHumanSupportButtonInBotPage=true`;
} else {
url = `${url}&showConversationMoment=always&conversationIntent=human&welcomeMessage=This is a welcome message for FAQ`;
}
window.open(url);
})();
</script>
# Page Example
Page examples based on the above scenario are as follows:
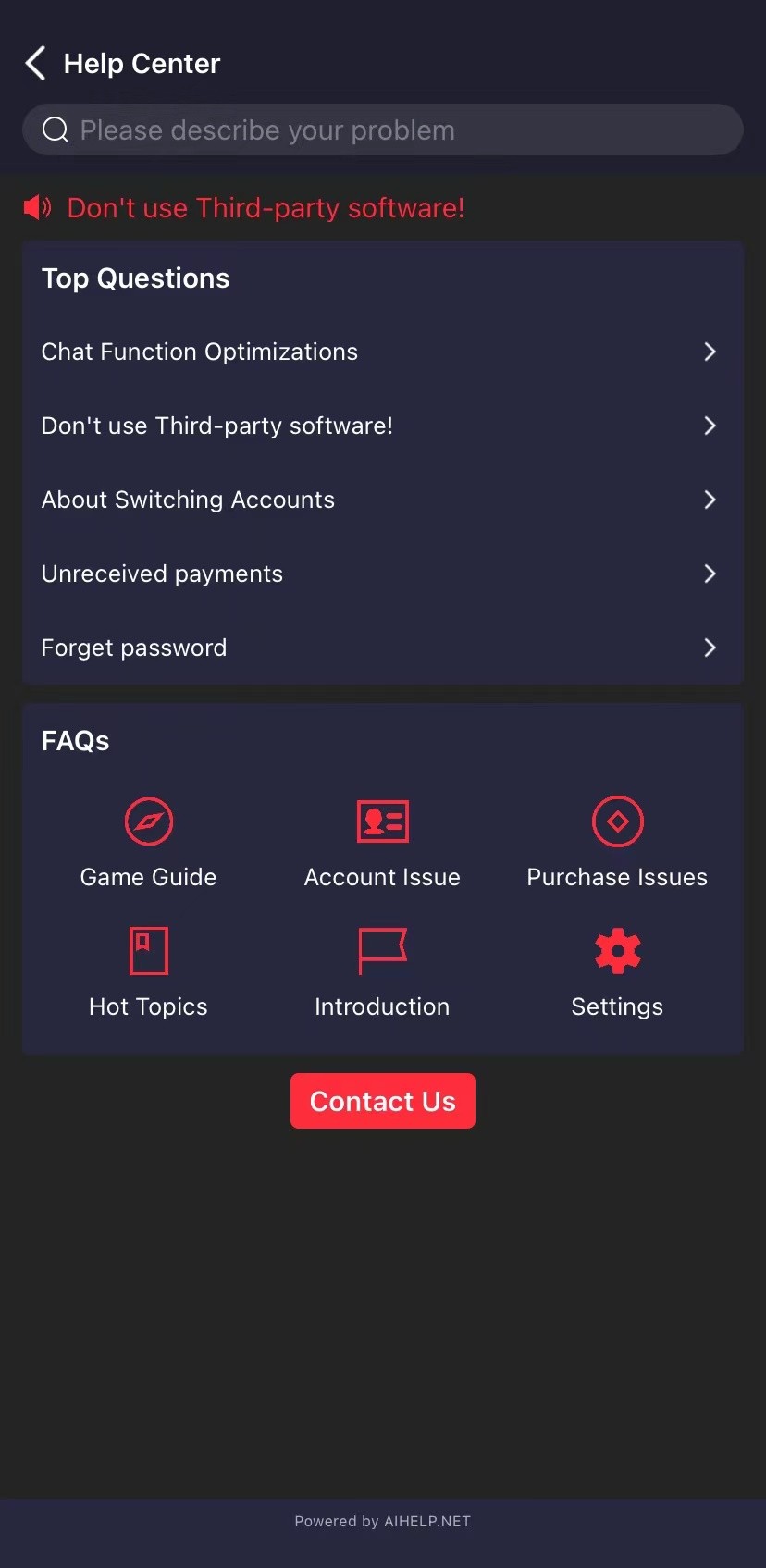
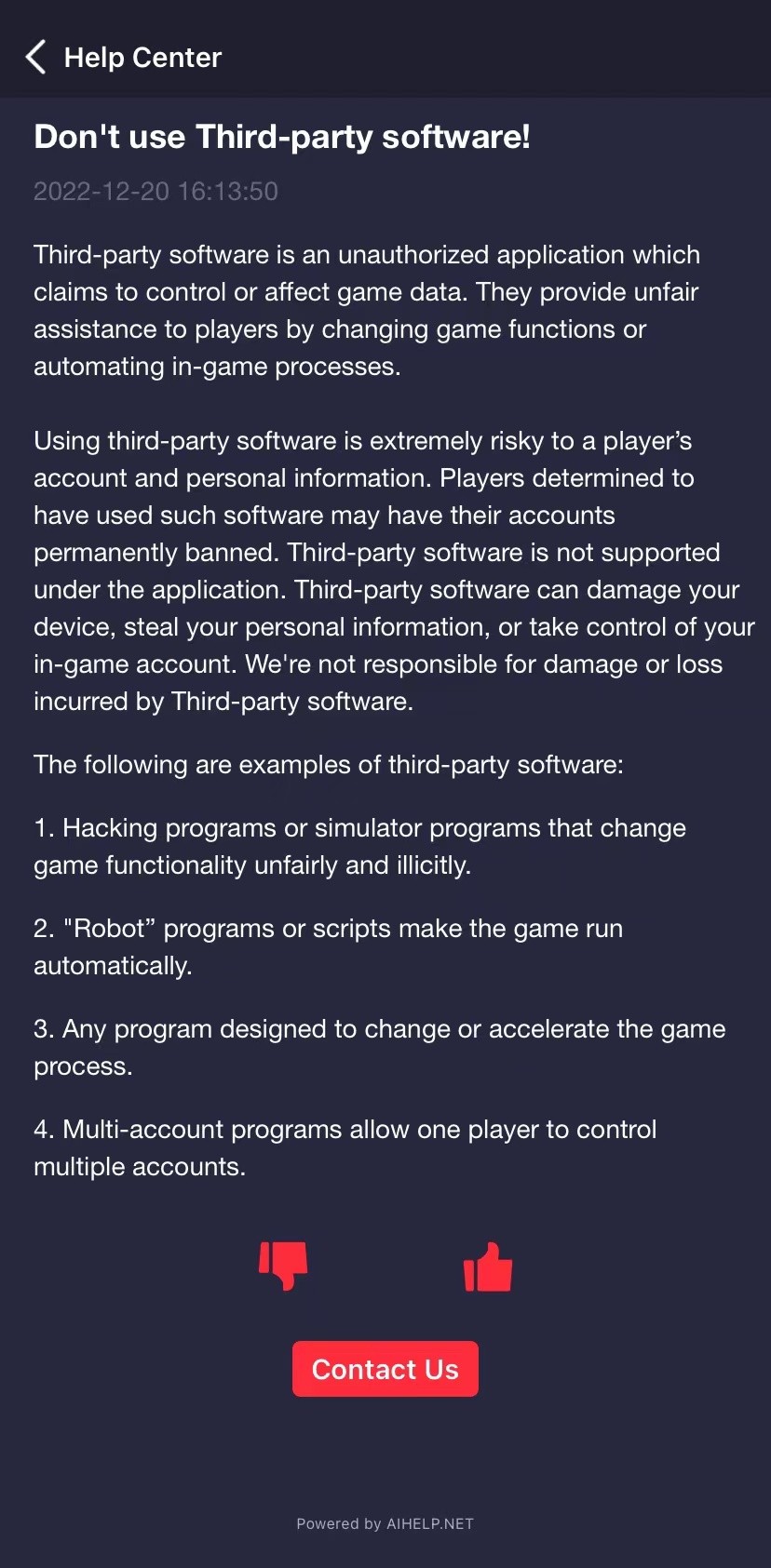
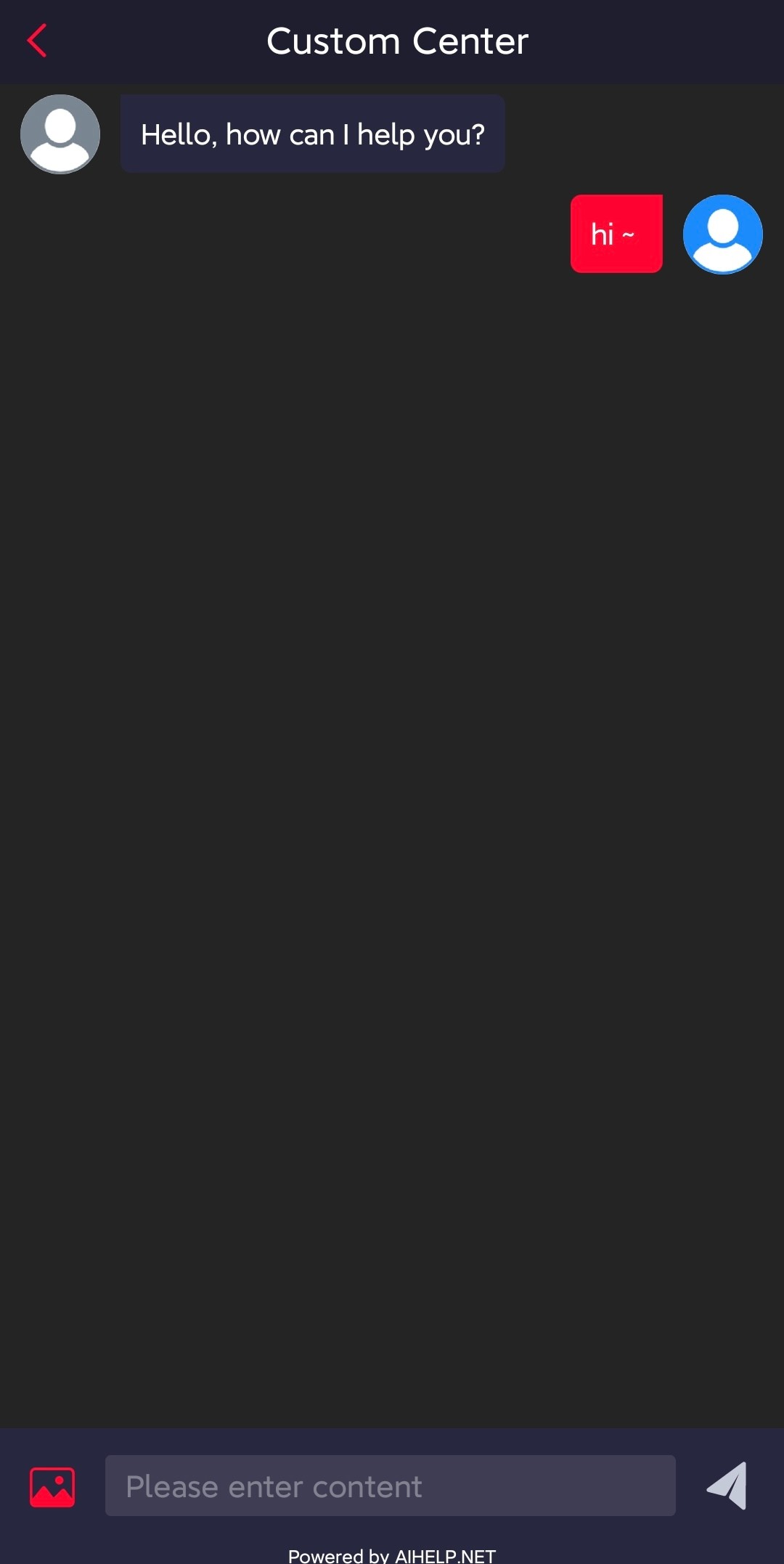