# Operation
We integrate announcements, guides, news, etc. with the customer service system at the same entrance, greatly improving the efficiency of information dissemination, so that users can understand the latest product trends more conveniently, and communicate with the customer service team more directly and efficiently.
# API
You could set the supportMode
property as showOperation
to notify AIHelp that you want to display the operation module:
<script src="https://cdn.aihelp.net/webchatv3/aihelp.js"></script>
<body>
<script>
(function () {
let initConfig = {
appKey: "THIS IS YOUR APP KEY",
domain: "THIS IS YOUR APP DOMAIN",
appId: "THIS IS YOUR APP ID",
supportMode: 'showOperation'
};
AIHelpSupport.init(initConfig);
AIHelpSupport.show();
})();
</script>
</body>
Or, you could make your own configuration about AIHelp via the supportConfig
property like this:
<script src="https://cdn.aihelp.net/webchatv3/aihelp.js"></script>
<body>
<script>
(function () {
let initConfig = {
appKey: "THIS IS YOUR APP KEY",
domain: "THIS IS YOUR APP DOMAIN",
appId: "THIS IS YOUR APP ID",
supportMode: 'showOperation',
supportConfig: {
selectIndex: 2,
conversationTitle: 'Hi, there',
alwaysShowHumanSupportButtonInBotPage: true,
welcomeMessage: 'This is a welcome message for operation'
}
};
AIHelpSupport.init(initConfig);
AIHelpSupport.show();
})();
</script>
</body>
# URL
Or, you could implement the same function by the URL scheme:
<script>
let appKey = "THIS IS YOUR APP KEY";
let domain = "THIS IS YOUR APP DOMAIN";
let appId = "THIS IS YOUR APP ID";
(function () {
let baseUrl = `https://${domain}/webchatv3/#/appKey/${appKey}/domain/${domain}/appId/${appId}`;
let params = `supportMode=showOperation&selectIndex=2&conversationTitle=Hi, there&alwaysShowHumanSupportButtonInBotPage=true&welcomeMessage=This is a welcome message for operation`;
let url = `${baseUrl}?${params}`;
window.open(url);
})();
</script>
# Definition
# supportMode
- Type:
showOperation
- Detail: Required. Support mode for AIHelp, set as
showOperation
to open up customer service.
# supportConfig API
- Type:
object
- Default:
{}
- Detail: Optional. Only works in API, customize configuration for AIHelp.
# selectIndex
- Type:
int
- Default:
0
- Detail: Optional. The default selected tab index.
# conversationTitle
- Type:
string
- Default:
HELP
- Detail: Optional. The title for customer service in Operation module.
# conversationIntent
- Type:
bot | human
- Default:
bot
- Detail: Optional. Intent for customer service display,
bot
for robot service,human
for human service.
# alwaysShowHumanSupportButtonInBotPage
- Type:
boolean
- Default:
false
- Detail: Optional. Entrance visibility for human support button in bot page.
# welcomeMessage
- Type:
string
- Default:
''
- Detail: Optional. Custom welcome message for human support.
# storyNode
- Type:
string
- Default:
''
- Detail: Optional. Entrance node for specific story line, a.k.a: story lines' user say.
# Scenario
Let’s assume a scenario where the application has different support and care schemes for different levels of users.
1、For users with level > 50, conversation tab is selected by default, and configure their conversation title to "Support";
2、At the same time, these users can choose to contact the manual customer service directly, and there is a special welcome message for them.
Then, the code example for this scenario is as follows:
# API
<script src="path/to/your/aihelp.js"></script>
<script>
(function (level) {
var supportConfig = {};
if (level > 50) {
supportConfig["selectIndex"] = Number.MAX_VALUE;
supportConfig["conversationTitle"] = "Support";
supportConfig["alwaysShowHumanSupportButtonInBotPage"] = true;
supportConfig["welcomeMessage"] = "This is a welcome message for operation";
} else {
supportConfig["selectIndex"] = 0;
}
var initConfig = {
appKey: "THIS IS YOUR APP KEY",
domain: "THIS IS YOUR APP DOMAIN",
appId: "THIS IS YOUR APP ID",
appName: "THIS IS YOUR APP NAME(OPTIONAL)",
language: "THIS IS YOUR DEFAULT LANGUAGE(OPTIONAL)",
supportMode: "showOperation",
supportConfig: supportConfig,
};
AIHelpSupport.init(initConfig);
AIHelpSupport.show();
})();
</script>
# URL
<script>
let appKey = 'THIS IS YOUR APP KEY';
let domain = 'THIS IS YOUR APP DOMAIN';
let appId = 'THIS IS YOUR APP ID';
(function (level) {
let url = `https://${domain}/webchatv3/#/appKey/${appKey}/domain/${domain}/appId/${appId}?supportMode=showOperation`;
if (level > 20 && level < 50) {
url = `${url}&selectIndex=3&conversationTitle=Support&alwaysShowHumanSupportButtonInBotPage=true&welcomeMessage=This is a welcome message for operation`;
} else if (level > 50) {
url = `${url}&selectIndex=0`;
}
window.open(url);
})();
</script>
# Page Example
Page examples based on the above scenario are as follows:
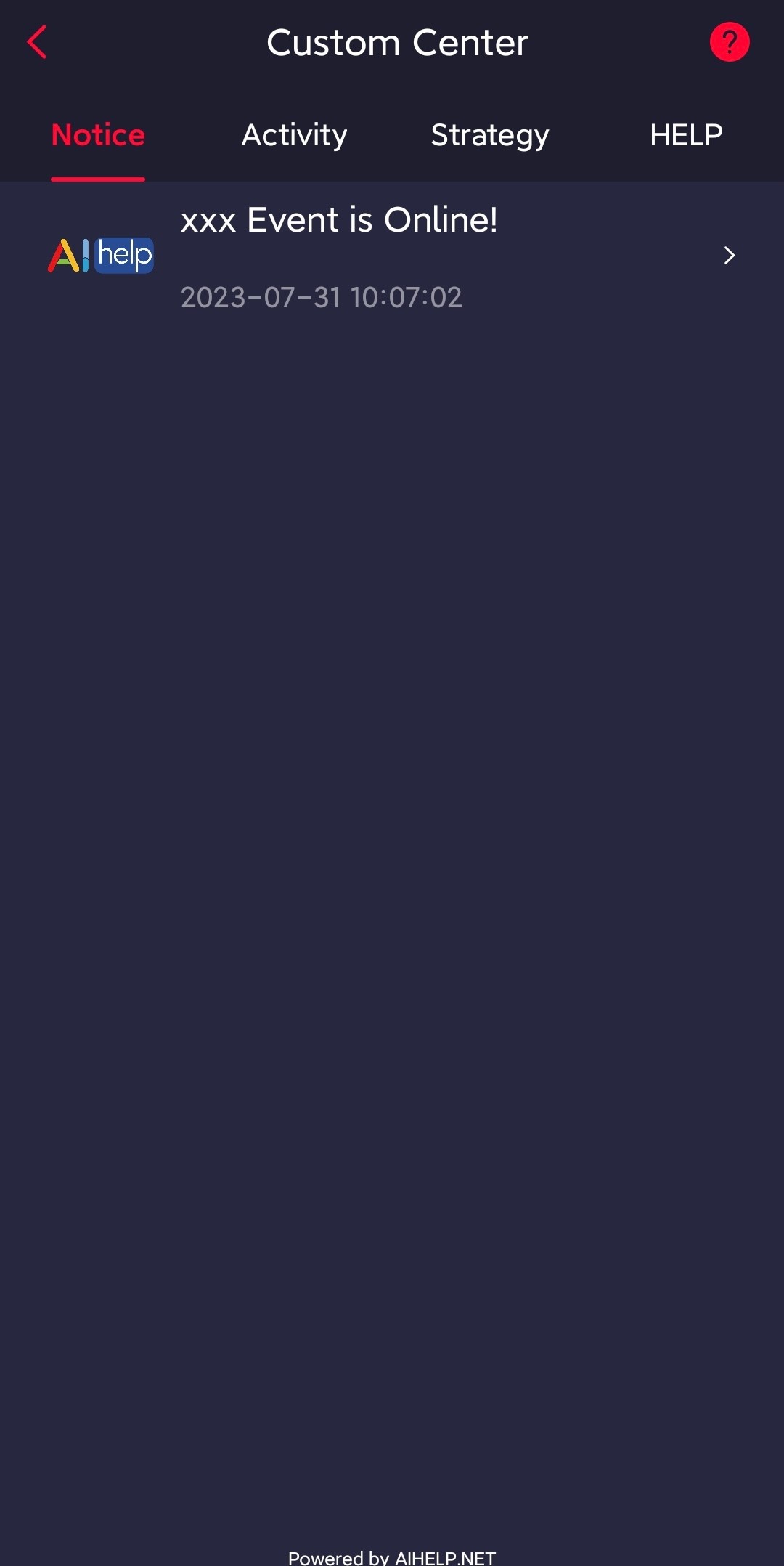
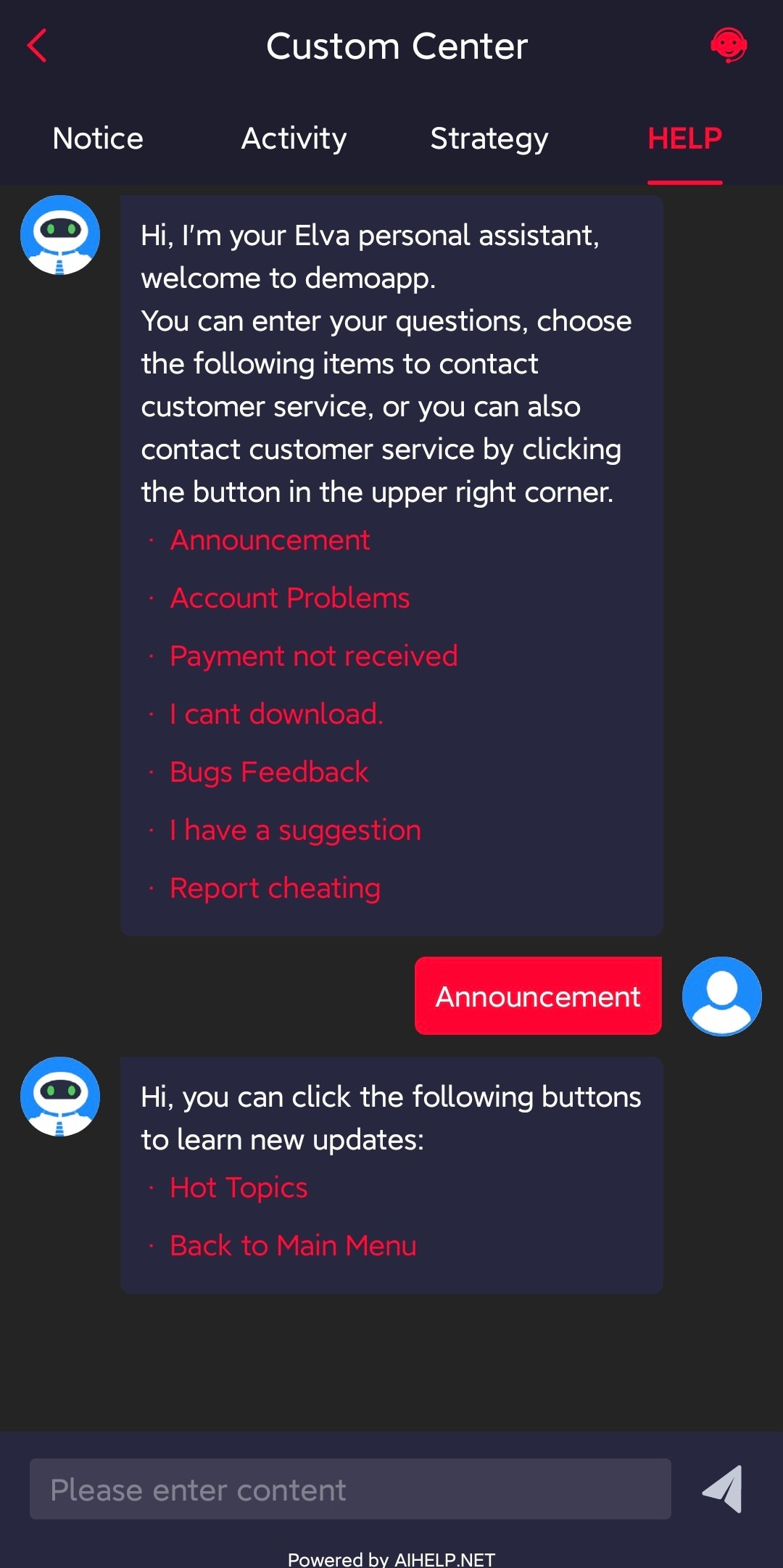
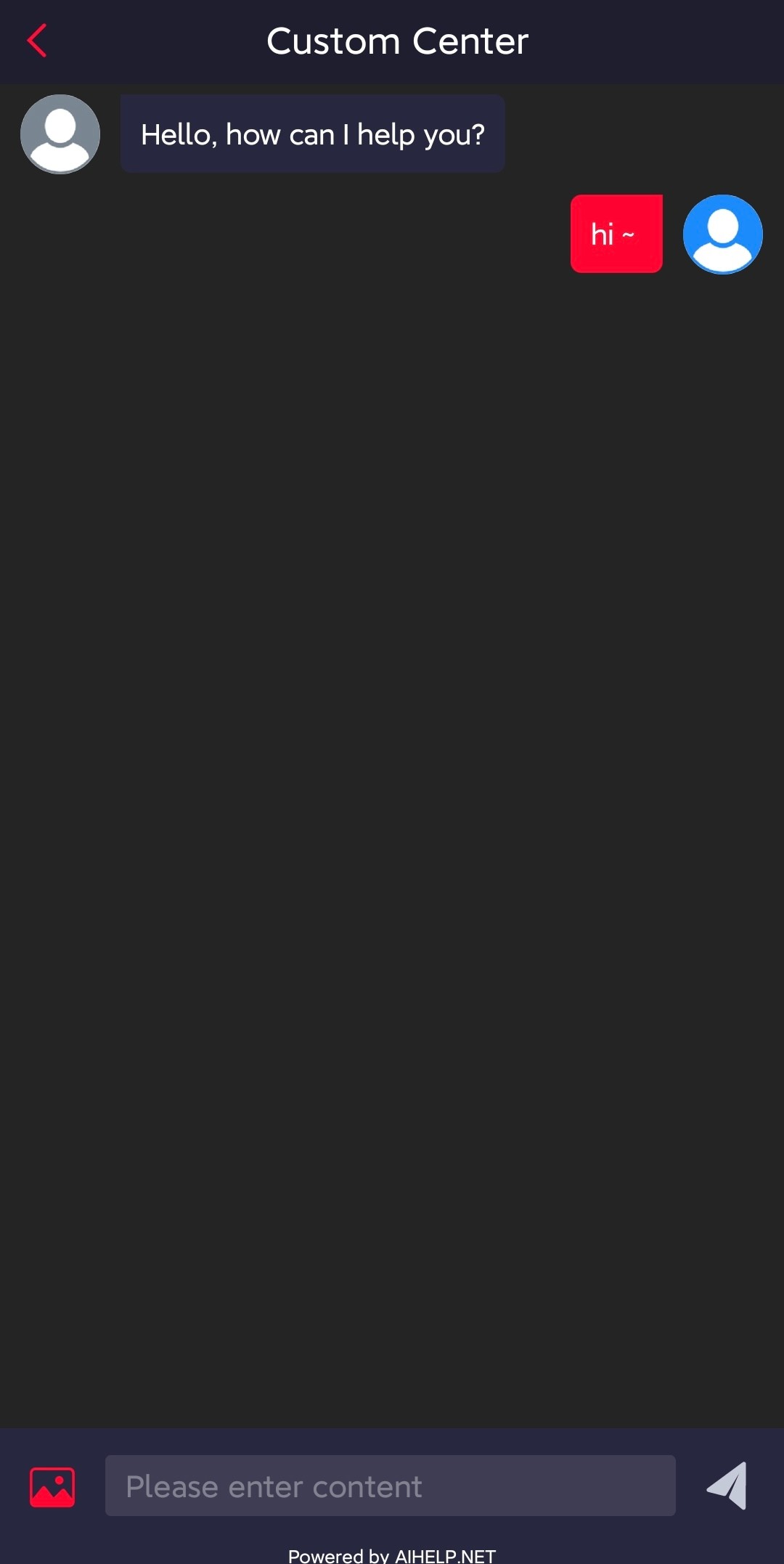
← FAQ Update Language →